acm The Least Palindromic Number
来源:学生作业帮 编辑:神马作文网作业帮 分类:综合作业 时间:2024/11/20 23:04:35
acm The Least Palindromic Number
Description
Palindromic numbers are digital strings that read the same both forwards and backwards. For example, 121, 44 and 3 are Palindromic numbers, 175, 36 are not;
For a given integer N, you are to find a palindromic number P that satisfy P>N. However, there are many such palindromic numbers. Your task is to find the least one.
Input
There are several test cases, each test case contains only one positive integer N in one line. The number of digits of N is not exceeding 10,000, and N has not lead zeroes.
The input will finish with the end of file.
Output
For each the case, your program will output the least palindromic number P (P > N) on separate line.
Sample Input
44
3
175
Sample Output
55
4
181
求高手解答.
Description
Palindromic numbers are digital strings that read the same both forwards and backwards. For example, 121, 44 and 3 are Palindromic numbers, 175, 36 are not;
For a given integer N, you are to find a palindromic number P that satisfy P>N. However, there are many such palindromic numbers. Your task is to find the least one.
Input
There are several test cases, each test case contains only one positive integer N in one line. The number of digits of N is not exceeding 10,000, and N has not lead zeroes.
The input will finish with the end of file.
Output
For each the case, your program will output the least palindromic number P (P > N) on separate line.
Sample Input
44
3
175
Sample Output
55
4
181
求高手解答.
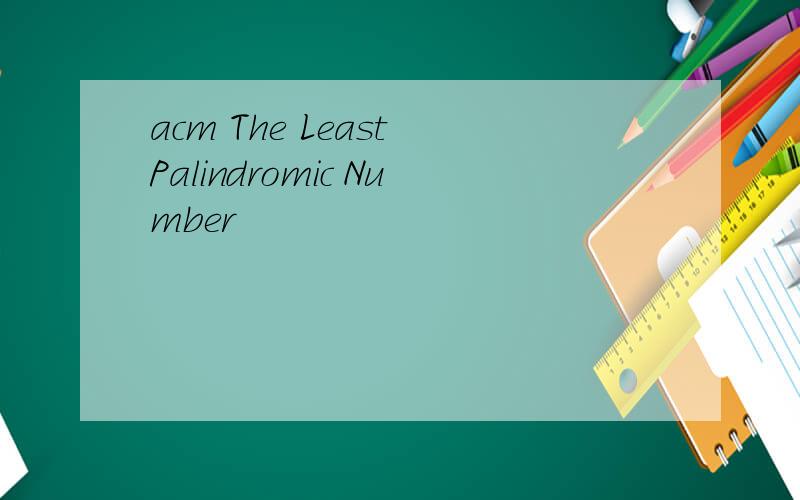
#include"stdio.h"
#include"string.h"
#include
using namespace std;
const int MAX=10005;
char s[MAX];
struct BigNum
{
int dig[MAX];
int len;
void Clr()
{
memset(dig,0,sizeof(dig));
len=1;
}
void print()
{
int i;
for(i=len-1;i>=0;i--)printf("%d",dig[i]);
}
};
BigNum GetN(char s[])
{
BigNum ret;
int i,j=0;
ret.Clr();
ret.len=strlen(s);
for(i=ret.len-1;i>=0;i--,j++)
ret.dig[j]=s[i]-'0';
return ret;
}
int cmp(BigNum a,BigNum b)
{
int i;
for(i=a.len-1;i>=0;i--)if(a.dig[i]!=b.dig[i])return a.dig[i]-b.dig[i];
return 0;
}
void GetHalf(BigNum ret)
{
int s,i,n=ret.len;
int len=ret.len,j;
BigNum x;
x.Clr();
if(ret.len%2==0)
{
x=ret;
s=n/2;
i=s;
for(j=i-1;j>=0;j--,i++)x.dig[j]=ret.dig[i];
if(cmp(x,ret)>0)
{
x.print();
return;
}
i=s;
ret.dig[s]++;
while(ret.dig[i]>9)
{
ret.dig[i+1]++;
ret.dig[i]-=10;
i++;
}
if(ret.dig[ret.len]>0)
{
ret.len++;
printf("1");
i=ret.len-2;
while(i>0)
{
putchar('0');
i--;
}
printf("1");
}
else
{
for(i=len-1;i>=s;i--)printf("%d",ret.dig[i]);
for(i=s;i=0;j--,i++)x.dig[j]=ret.dig[i];
if(cmp(x,ret)>0)
{
x.print();
return;
}
i=s;
ret.dig[s]++;
while(ret.dig[i]>9)
{
ret.dig[i+1]++;
ret.dig[i]-=10;
i++;
}
if(ret.dig[ret.len]>0)
{
ret.len++;
printf("1");
i=ret.len-2;
while(i>0)
{
putchar('0');
i--;
}
printf("1");
}
else
{
for(i=len-1;i>=s;i--)printf("%d",ret.dig[i]);
for(i=s+1;i
再问: 能不能精简一些?
再答: 我不想改了,能做出来已经不错了。你自己看看能不能哪里合并一下吧。总的思路就是先把本来的数字对称处理一下看看是不是比原来的大,如果是就输出 如果不是的话就把中间的一位加1,再比一比,如果进位了,就输出100001 这个样子的数字,位数是原来的位数加1 如果没有进位就对称一下输出
#include"string.h"
#include
using namespace std;
const int MAX=10005;
char s[MAX];
struct BigNum
{
int dig[MAX];
int len;
void Clr()
{
memset(dig,0,sizeof(dig));
len=1;
}
void print()
{
int i;
for(i=len-1;i>=0;i--)printf("%d",dig[i]);
}
};
BigNum GetN(char s[])
{
BigNum ret;
int i,j=0;
ret.Clr();
ret.len=strlen(s);
for(i=ret.len-1;i>=0;i--,j++)
ret.dig[j]=s[i]-'0';
return ret;
}
int cmp(BigNum a,BigNum b)
{
int i;
for(i=a.len-1;i>=0;i--)if(a.dig[i]!=b.dig[i])return a.dig[i]-b.dig[i];
return 0;
}
void GetHalf(BigNum ret)
{
int s,i,n=ret.len;
int len=ret.len,j;
BigNum x;
x.Clr();
if(ret.len%2==0)
{
x=ret;
s=n/2;
i=s;
for(j=i-1;j>=0;j--,i++)x.dig[j]=ret.dig[i];
if(cmp(x,ret)>0)
{
x.print();
return;
}
i=s;
ret.dig[s]++;
while(ret.dig[i]>9)
{
ret.dig[i+1]++;
ret.dig[i]-=10;
i++;
}
if(ret.dig[ret.len]>0)
{
ret.len++;
printf("1");
i=ret.len-2;
while(i>0)
{
putchar('0');
i--;
}
printf("1");
}
else
{
for(i=len-1;i>=s;i--)printf("%d",ret.dig[i]);
for(i=s;i=0;j--,i++)x.dig[j]=ret.dig[i];
if(cmp(x,ret)>0)
{
x.print();
return;
}
i=s;
ret.dig[s]++;
while(ret.dig[i]>9)
{
ret.dig[i+1]++;
ret.dig[i]-=10;
i++;
}
if(ret.dig[ret.len]>0)
{
ret.len++;
printf("1");
i=ret.len-2;
while(i>0)
{
putchar('0');
i--;
}
printf("1");
}
else
{
for(i=len-1;i>=s;i--)printf("%d",ret.dig[i]);
for(i=s+1;i
再问: 能不能精简一些?
再答: 我不想改了,能做出来已经不错了。你自己看看能不能哪里合并一下吧。总的思路就是先把本来的数字对称处理一下看看是不是比原来的大,如果是就输出 如果不是的话就把中间的一位加1,再比一比,如果进位了,就输出100001 这个样子的数字,位数是原来的位数加1 如果没有进位就对称一下输出
acm The Least Palindromic Number
the least possible number?
27 is the least number of the numbers49,38,27
1、The least number natural number whose digits sum equals 10
英语翻译31.In the table above,what is the least number of table
亚里士多德的 “What is common to the greatest number gets the least
the least
what is the least number of points at which p can intersect
ACM B-number 题目意思解释
杭电ACM 1019Problem DescriptionThe least common multiple (LCM)
acm The Drunk Jailer
acm Calculate the Sum